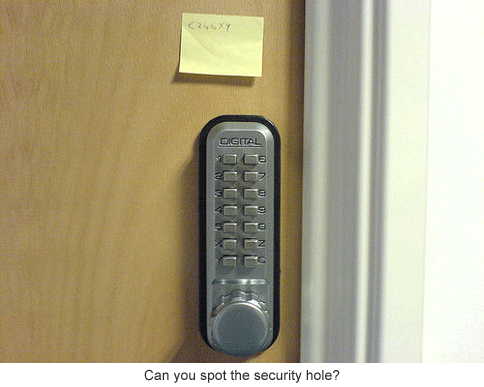
I have explained in The Three Steps of Building an ASP.NET Validator Control, how to build a validator control from the ground up in three easy steps and in a reusable format. I highly recommend reading it before going any further.
Here I am discussing the common validator control security holes that might compromise your forms security when left untreated.
Security Hole 1: Failing to Implement The Server-Side Validation
When building a validator control from scratch or using a CustomValidator
, you should always implement server-side validation (i.e. the .NET code), however, the client-side validation (i.e. The javascript) code is optional but nice to have.
The reason is, a malicious user might disable JavaScript from his browser to bypass your validation or might not even be using a browser but some code to post to your form.
Always start by building the server-side validation, test it, then start building the client one.
Security Hole 2: Failing to check Page.IsValid
This is a major pitfall and failing to avoid it will render your validation next to useless! Before you process any data coming from your form, you need to make sure that it has passed your validators, however, if the client’s browser has JavaScript disabled, then the code will reach the server without validation and will require you to check the Page.IsValid
manually. For example:
protected void Submit_Click(object sender, EventArgs e) {
// Very Important
if (!Page.IsValid) {
return;
}
// Do some processing here...
}
Security Hole 3: Client-side and Server-Side Regular Expressions
Regular expressions AKA RegEx on the server-side and the client-side may not have the same desired effect, in other words, the same regular expression syntax that works on the server side, might not work on the client side. So make sure you thoroughly test both.
Security Hole 4: Relying on the MaxLength
property of a TextBox
This is not directly related to validator controls, however, it belongs to validation and I thought of mentioning it for completion.
You should not rely only on MaxLength
to restrict the max allowed text for your TextBox
as this property can be easily bypassed on the client, by setting the TextBox
with JavaScript for example, and will never be checked on the server-side. However, set it anyway for usability purpose.
To force a max length on a TextBox
, you might use a RegularExpressionValidator
with the validation expression ^.{0,MaxLength}$
.
Always test your validators in both modes JavaScript enabled and disabled. To turn off JavaScript in Internet Explorer, you can go to the “Security Settings”, however, I recommend using Internet Explorer Developer Toolbar</a>.
If you think that there are more security holes that are worth mentioning then please drop me a comment line.